Switch / SwitchCompat
Switch là loại View, mở rộng từ CompoundButton, nên nó có các thiết lập, thuộc tính, triển khai code và XML tương tự như TextView, Button, CheckBox nên bạn có thể tham khảo các nội dung đó trước.
Loại View này cho phép bật tắt qua lại giữa hai trạng thái bằng cách bấm, hoặc kéo một cái công tắc gạt (thumb) trượt trên một đường ngắn (track). Có hai phiên bản Switch (android.widget.Switch) và SwitchCompat (android.support.v7.widget.SwitchCompat), để đảm bảo tính năng hiện đại tương thích trên nhiều thiết bị khi sử dụng Library Support nên sử dụng SwitchCompat
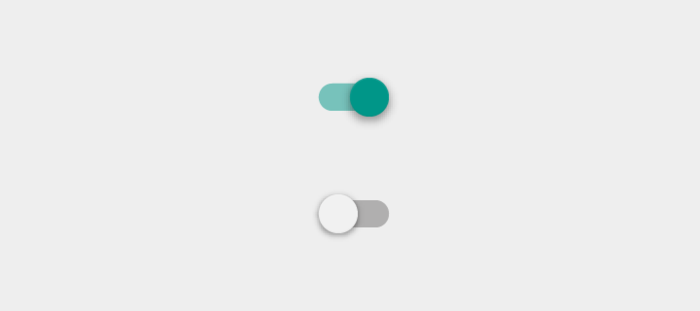
Triển khai XML SwitchCompat
<android.support.v7.widget.SwitchCompat android:id="@+id/switch_id" android:text="Test Switch" android:layout_width="wrap_content" android:layout_height="wrap_content" />
Code Java xử Switch
Cách viết code giống với CheckBox với các phương thức như:
isChecked
setChecked
setOnCheckedChangeListener
setOnClickListener
Ví dụ Switch/SwitchCompat
layout/layout_mainactivity.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:orientation="vertical" android:padding="40dp" android:layout_width="match_parent" android:layout_height="match_parent"> <android.support.v7.widget.SwitchCompat android:id="@+id/switch_id" android:text="Test Switch" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <TextView android:id="@+id/textview_id" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </LinearLayout>
MainActivity.java
public class MainActivity extends AppCompatActivity { TextView textView; SwitchCompat switchCompat; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.layout_mainactivity); textView = findViewById(R.id.textview_id); switchCompat = findViewById(R.id.switch_id); switchCompat.setOnCheckedChangeListener( new CompoundButton.OnCheckedChangeListener() { @Override public void onCheckedChanged(CompoundButton compoundButton, boolean b) { textView.setText("Switch is " + (switchCompat.isChecked() ? "On" : "Off")); } }); } }
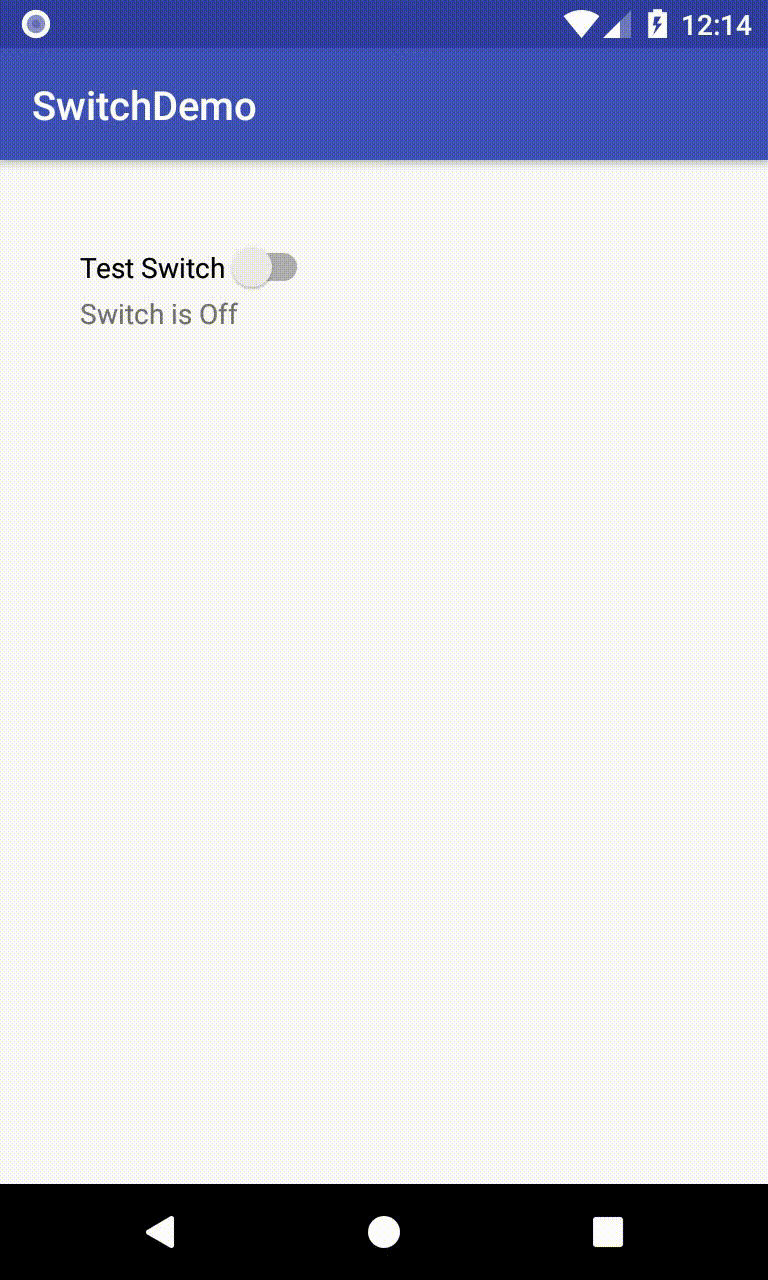
Một số thiết lập
-
Thiết lập dòng text: on / off
Trong XML
android:textOn="Bật" android:textOff="Tắt" app:showText="true"
Hoặc trong Code Java
switchCompat.setTextOff("Tắt"); switchCompat.setTextOn("Bật"); switchCompat.setShowText(true);
-
Thay đổi độ rộng phần trượt
Trong XML thiết lập: app:switchMinWidth="100dp" hoặc Java thay đổi độ rộng (theo pixel) switchCompat.setSwitchMinWidth(150);
-
Màu của Thumb và Track trong Switch
Trong XML sử dụng thuộc tính app:thumbTint, app:trackTint để thiết lập màu, có thể gán màu trực tiếp ví dụ như:
app:thumbTint="#0d47a1" app:trackTint="#cc1e1e"
Việc gán màu trực tiếp thì làm cho màu của Thumb, Track không có sự thay đổi giữa trạng thái bật và tắt. Nếu muốn thiết lập màu cho cả ở trạng thái bật, tắt thì không gán giá trị màu trực tiếp mà gán giá trị Selector với cấu trúc như ví dụ:
Có một Selector
drawable/switch_thumb.xml
<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <!--Màu ở trạng thái bật--> <item android:state_checked="true" android:color="#38ae20" /> <!--Màu ở trạng thái bình thường (tắt) --> <item android:color="#d9c9c9" /> </selector>
Tương tự tạo ra một Selector cho track:
drawable/switch_track.xml
<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <item android:state_checked="true" android:color="#80967b" /> <item android:color="#464141" /> </selector>
Gán vào Switch ở XML bằng cách
app:thumbTint="@drawable/switch_thumb" app:trackTint="@drawable/switch_track"
Kết quả tương tự khi dùng code Java với phương thức setThumbTintList, setTrackTintList với tham số là đối tượng ColorStateList
ColorStateList colorThumb = new ColorStateList( new int[][] { new int[] {android.R.attr.state_checked}, //trạng thái checked new int[] {} //trạng thái bình thường }, new int[] { Color.RED, //Màu tương ứng checked Color.GRAY //Màu ở trạng thái bình thường } ); switchCompat.setThumbTintList(colorThumb);
-
Gán Drawable vào Thumb và Track
Tùy chọn Drawable cho track dùng thuộc tính app:track, và gán Drawable cho thumb dùng thuộc tính android:thumb
Ví dụ có Drawable
@drawable/switch_mytrack.xml
(vẽ một hình chữ nhật cao 30dp)<?xml version="1.0" encoding="utf-8"?> <layer-list xmlns:android="http://schemas.android.com/apk/res/android"> <item android:gravity="center_vertical|fill_horizontal" android:start="4dp" android:end="4dp"> <shape android:shape="rectangle" android:tint="#71c25b"> <corners android:radius="10dp" /> <solid android:color="#707c70" /> <size android:height="30dp" /> </shape> </item> </layer-list>
Có drawable
drawable/switch_mythumb.xml
(vẽ một hình vuông 60x60dp)<?xml version="1.0" encoding="utf-8"?> <layer-list xmlns:android="http://schemas.android.com/apk/res/android"> <item android:width="60dp" android:height="60dp"> <shape android:shape="rectangle"> <solid android:color="#963e3e" /> </shape> </item> </layer-list>
Gán nó vào Switch
app:track="@drawable/switch_mytrack" android:thumb="@drawable/switch_mythumb"