CompoundButton
CompoundButton là một lớp cơ abstract mở rộng từ TextView (Button), từ lớp này nó được mở rộng để xây dựng các View là: CheckBox, RadioButton, Switch, ToggleButton
Các lớp mở rộng CheckBox, RadioButton, Switch, ToggleButton lưu lại các thuộc tính, ứng xử tương tự như TextView, Button nhưng có thêm hai trạng thái là checked
và unchecked
Một số thuộc tính, phương thức chung cho CheckBox, RadioButton, Switch, ToggleButton
Trong XML
- android:checked thiết lập trạng thái checked, unchecked với giá trị "true" hay "false"
- android:button để gán Drawable vào View (vẽ trạng thái cho CheckBox, RadioButton ...)
- android:buttonTint để gán màu Tint
Trong code Java
- isChecked() để kiểm tra trạng thái là checked (true) hay unchecked (false)
- setOnCheckedChangeListener bắt sự kiện khi trạng thái (checked/unchecked) chuyển đổi:
button.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() { @Override public void onCheckedChanged(CompoundButton compoundButton, boolean b) { //b - trạng thái //code làm gì khi chuyển trạng thái ở đây } });
CheckBox
Lưu ý khi sử dụng CheckBox nó có các thuộc tính tương tự TextView, Button
Triển khai CheckBox trong XML
<CheckBox android:id="@+id/checkbox_id" android:text="Java - Android" android:checked="true" <!--Hoặc "false" --> android:layout_width="wrap_content" android:layout_height="wrap_content" />
Code Java
final CheckBox checkBox = findViewById(R.id.checkbox_id); //Kiểm tra checked if (checkBox.isChecked()) { //Checked } else { //Unchecked } //Thiết lập trạng thái check boolean toi_chon = true; checkBox.setChecked(toi_chon); //Bắt sự kiện thay đổi trạng thái checkBox.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() { @Override public void onCheckedChanged(CompoundButton compoundButton, boolean b) { //Code khi trạng thái check thay đổi Toast.makeText( compoundButton.getContext(), compoundButton.getText()+"|"+b, Toast.LENGTH_SHORT).show(); } }); //Bắt sự kiện Click checkBox.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Toast.makeText( view.getContext(), "Click!", Toast.LENGTH_SHORT).show(); } });
Ví dụ về CheckBox
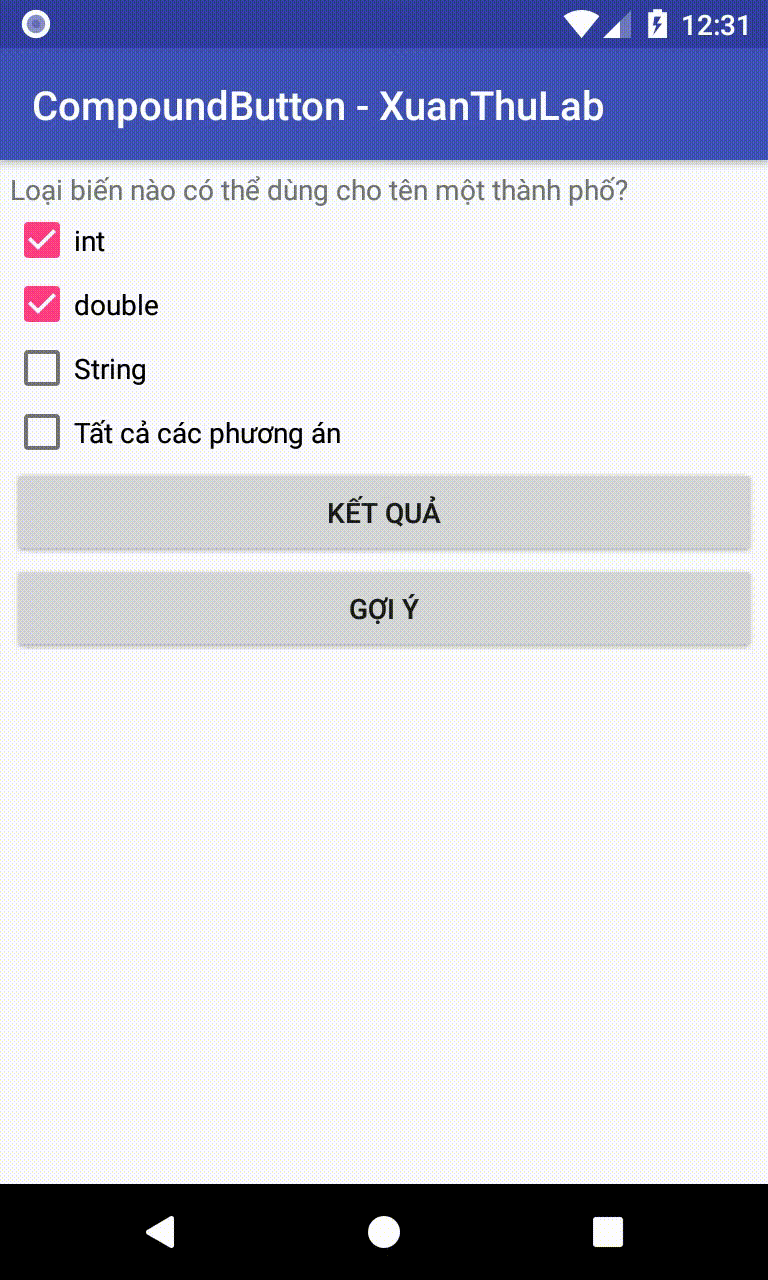
layout/activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:padding="5dp" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:text="Loại biến nào có thể dùng cho tên một thành phố?" android:layout_width="match_parent" android:layout_height="wrap_content" /> <CheckBox android:id="@+id/int_id" android:text="int" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <CheckBox android:id="@+id/double_id" android:text="double" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <CheckBox android:id="@+id/string_id" android:text="String" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <CheckBox android:id="@+id/all" android:text="Tất cả các phương án" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <Button android:id="@+id/test" android:text="Kết quả" android:layout_width="match_parent" android:layout_height="wrap_content" /> <Button android:id="@+id/hint" android:text="Gợi ý" android:layout_width="match_parent" android:layout_height="wrap_content" /> </LinearLayout>
MainActivity.java
public class MainActivity extends AppCompatActivity { CheckBox int_id, double_id, string_id, all; Button test, hint; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); init(); } //Listener nhận sự kiện khi các Checkbox thay đổi trạng thái CompoundButton.OnCheckedChangeListener m_listener = new CompoundButton.OnCheckedChangeListener() { @Override public void onCheckedChanged(CompoundButton compoundButton, boolean b) { if (compoundButton == all) { detachListener(); int_id.setEnabled(!b); double_id.setEnabled(!b); string_id.setEnabled(!b); int_id.setChecked(b); double_id.setChecked(b); string_id.setChecked(b); attachListener(); } else { Toast.makeText(compoundButton.getContext(), compoundButton.getText() + " | " + compoundButton.isChecked(), Toast.LENGTH_SHORT).show(); } } }; //Gán Listener vào CheckBox void attachListener() { int_id.setOnCheckedChangeListener(m_listener); double_id.setOnCheckedChangeListener(m_listener); string_id.setOnCheckedChangeListener(m_listener); all.setOnCheckedChangeListener(m_listener); } //Bỏ các Listener khỏi CheckBox void detachListener() { int_id.setOnCheckedChangeListener(null); double_id.setOnCheckedChangeListener(null); string_id.setOnCheckedChangeListener(null); all.setOnCheckedChangeListener(null); } void init() { int_id = findViewById(R.id.int_id); double_id = findViewById(R.id.double_id); string_id = findViewById(R.id.string_id); all = findViewById(R.id.all); attachListener(); test = findViewById(R.id.test); hint = findViewById(R.id.hint); hint.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { detachListener(); int_id.setChecked(false); double_id.setChecked(false); all.setChecked(false); attachListener(); string_id.setChecked(true); } }); test.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { String mgs = ""; if (!int_id.isChecked() && !double_id.isChecked() && string_id.isChecked()) mgs = "Đúng, chúc mừng"; else mgs = "Sai rồi"; Toast.makeText(view.getContext(), mgs, Toast.LENGTH_SHORT).show(); } }); } }
Một số tùy biến với CheckBox
-
Tùy chọn hình vẽ CheckBox với android:draw
Giả sử có 2 tài nguyên hình ảnh vẽ trạng thái check và uncheck:
và
với tên
drawable/checkbox_empty
vàdrawable/checkbox_check
lúc đó có thể tạo ra một selector như saudrawable/checkbox_custome.xml
<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <item android:state_checked="false" android:drawable="@drawable/checkbox_empty" /> <item android:state_checked="true" android:drawable="@drawable/checkbox_check" /> </selector>
Gán cho CheckBox muốn sử dụng selector này như sau:
android:button="@drawable/checkbox_custome"